2024. 6. 5. 10:55ㆍHigh Level Programming Language/Learning the Java Language
Numbers
이 섹션은 Number 클래스(java.lang 패키지에 있음)와 그 하위 클래스에 대한 논의로 시작됩니다. 특히 이 섹션에서는 기본 데이터 유형 대신 이러한 클래스의 인스턴스화를 사용하는 상황에 대해 설명합니다. 또한 이 섹션에서는 자바 언어에 내장된 연산자를 보완하기 위해 서식을 지정하거나 수학 함수를 사용하는 등 숫자 작업에 필요할 수 있는 클래스들에 대해 설명합니다. 마지막으로 코드를 단순화하는 컴파일러 기능인 오토박싱 및 언박싱에 대한 논의가 있습니다.
Strings
Java 프로그래밍에서 널리 사용되는 문자열은 일련의 문자입니다. Java 프로그래밍 언어에서 문자열은 객체입니다. 이 섹션에서는 String 클래스를 사용하여 문자열을 만들고 조작하는 방법을 설명합니다. 또한 String 및 StringBuilder 클래스를 비교합니다.
Numbers
이 섹션은 java.lang 패키지의 Number 클래스, 해당 하위 클래스 및 기본 숫자 유형 대신 이러한 클래스의 인스턴스화를 사용하는 상황에 대한 논의로 시작됩니다.
이 섹션에서는 형식화된 숫자 출력을 작성하기 위한 메서드를 제공하는 PrintStream 및 DecimalFormat 클래스도 제공합니다.
마지막으로 java.lang의 Math 클래스에 대해 설명합니다. 여기에는 언어에 내장된 연산자를 보완하는 수학 함수가 포함되어 있습니다. 이 클래스에는 삼각 함수, 지수 함수 등에 대한 메서드가 있습니다.
The Numbers Classes
숫자로 작업할 때 대부분의 경우 코드에서 기본[Primitive] 타입을 사용합니다. 예를 들어:
int i = 500;
float gpa = 3.65f;
byte mask = 0x7f;
그러나 기본 타입 대신 객체를 사용하는 이유가 있으며 Java 플랫폼은 각 기본 데이터 타입에 대한 래퍼 클래스를 제공합니다. 이러한 클래스는 객체의 기본 요소를 "래핑"합니다. 종종 래핑은 컴파일러에 의해 수행됩니다. 객체가 예상되는 곳에 기본 형식을 사용하는 경우 컴파일러는 래퍼 클래스에서 기본 형식을 박스에 넣습니다-이를 오토박싱이라함. 마찬가지로, 기본 요소가 필요할 때 숫자 객체를 사용하면 컴파일러가 객체를 unboxing합니다. 자세한 내용은 Autoboxing 및 Unboxing을 참조하세요.
모든 숫자 래퍼 클래스는 추상 클래스 Number의 하위 클래스입니다.
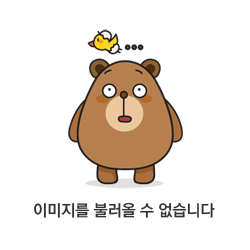
참고: 여기에서 논의되지 않은 Number의 다른 네 가지 하위 클래스가 있습니다. BigDecimal 및 BigInteger는 고정밀 계산에 사용됩니다. AtomicInteger 및 AtomicLong은 다중 스레드 애플리케이션에 사용됩니다.
기본 요소[primitives]가 아닌 Number 객체를 사용하는 세 가지 이유는 다음과 같습니다.
1. 객체를 기대하는 메소드의 아규먼트(종종 숫자 모음을 조작할 때 사용됨)
2. 데이터 유형의 상한 및 하한을 제공하는 MIN_VALUE 및 MAX_VALUE와 같이 클래스에서 정의한 상수를 사용합니다.
3. 값을 다른 기본 유형으로 변환하고, 문자열로 변환하고, 숫자 시스템(10진수, 8진수, 16진수, 2진수) 간 변환을 위해 클래스 메소드를 사용합니다.
다음 표에는 Number 클래스의 모든 하위 클래스가 구현하는 인스턴스 메서드가 나열되어 있습니다.
Number의 모든 하위 클래스에 의해 구현되는 메서드들
Method | Description |
byte byteValue() short shortValue() int intValue() long longValue() float floatValue() double doubleValue() |
이 Number 객체의 값을 리턴 기본 데이터 유형으로 변환합니다. |
int compareTo (Byte anotherByte ) int compareTo (Double anotherDouble ) int compareTo (Float anotherFloat ) int compareTo (Integer anotherInteger ) int compareTo (Long anotherLong ) int compareTo (Short anotherShort ) |
이 Number 객체를 아규먼와 비교합니다. |
boolean equals (Object obj) | 이 Number 객체가 아규먼트와 같은지 여부를 확인합니다. 아규먼트가 null이 아니고 동일한 타입 및 동일한 숫자 값을 갖는 객체인 경우 메서드는 true를 반환합니다. Java API 문서에 설명된 Double 및 Float 객체에 대한 몇 가지 추가 요구 사항이 있습니다. |
각 Number 클래스에는 숫자와 문자열 간 변환 및 숫자 체계 간 변환에 유용한 다른 메서드가 포함되어 있습니다. 다음 표에는 Integer 클래스의 이러한 메서드가 나열되어 있습니다. 다른 Number 하위 클래스에 대한 메서드도 비슷합니다.
Conversion Methods, Integer Class
Method | Description |
static Integer decode(String s) | Decodes a string into an integer. Can accept string representations of decimal, octal, or hexadecimal numbers as input. |
static int parseInt(String s) | Returns an integer (decimal only). |
static int parseInt(String s, int radix) | Returns an integer, given a string representation of decimal, binary, octal, or hexadecimal (radix equals 10, 2, 8, or 16 respectively) numbers as input. |
String toString() | Returns a String object representing the value of this Integer. |
static String toString(int i) | Returns a String object representing the specified integer. |
static Integer valueOf(int i) | Returns an Integer object holding the value of the specified primitive. |
static Integer valueOf(String s) | Returns an Integer object holding the value of the specified string representation. |
static Integer valueOf(String s, int radix) | Returns an Integer object holding the integer value of the specified string representation, parsed with the value of radix. For example, if s = "333" and radix = 8, the method returns the base-ten integer equivalent of the octal number 333. |
Formatting Numeric Print Output
앞에서 문자열을 표준 출력(System.out)으로 프린트하기 위해 print 및 println 메서드를 사용하는 것을 보았습니다. 모든 숫자는 문자열로 변환될 수 있으므로(이 단원의 뒷부분에서 살펴보겠지만) 이러한 메서드를 사용하여 문자열과 숫자의 임의 혼합을 프린트할 수 있습니다. 그러나 Java 프로그래밍 언어에는 숫자가 포함될 때 프린트 출력을 훨씬 더 효과적으로 제어할 수 있는 다른 방법이 있습니다.
The printf and format Methods
java.io 패키지에는 print 및 println을 대체하는 데 사용할 수 있는 두 가지 포맷 지정 방법이 있는 PrintStream 클래스가 포함되어 있습니다. 이러한 메소드인 format과 printf는 서로 동일합니다. 지금까지 사용했던 친숙한 System.out은 PrintStream 객체이므로 System.out에서 PrintStream 메서드를 호출할 수 있습니다. 따라서 이전에 print 또는 println을 사용했던 코드의 어느 곳에서나 format 또는 printf를 사용할 수 있습니다. 예를 들어,
System.out.format(.....);
이 두 가지 java.io.PrintStream 메소드의 신택스는 동일합니다.
public PrintStream format(String format, Object... args)
여기서 format은 사용할 포맷을 지정하는 문자열이고 args는 해당 포맷을 사용하여 프린트할 변수 목록입니다. 간단한 예는 다음과 같습니다.
System.out.format("The value of " + "the float variable is " +
"%f, while the value of the " + "integer variable is %d, " +
"and the string is %s", floatVar, intVar, stringVar);
첫 번째 파라미터인 format은 두 번째 파라미터인 args의 객체 형식을 지정하는 방법을 지정하는 포맷 문자열입니다. 포맷 문자열에는 Object...args의 아규먼트 형식을 지정하는 특수 문자인 포맷 지정자와 일반 텍스트가 포함됩니다. (Object...args 표기법을 varargs라고 하며 이는 아규먼트 개수가 다를 수 있음을 의미합니다.)
포맷 지정자는 백분율 기호(%)로 시작하고 컨버터(위 예제로는, f, d, s)로 끝납니다. 컨버터는 포맷화할 아규먼트의 타입을 나타내는 문자입니다. 백분율 기호(%)와 컨버터 사이에는 선택적 플래그와 지정자가 있을 수 있습니다. java.util.Formatter에 문서화되어 있는 많은 컨버터, 플래그 및 지정자가 있습니다.
다음은 기본 예입니다.
int i = 461012;
System.out.format("The value of i is: %d%n", i);
%d는 단일 변수가 10진 정수임을 지정합니다. %n은 플랫폼 독립적인 개행 문자입니다. 출력은 다음과 같습니다
The value of i is: 461012
printf 및 format 메소드가 오버로드되었습니다. 각각에는 다음 구문을 사용하는 버전이 있습니다.
public PrintStream format(Locale l, String format, Object... args)
예를 들어, 프랑스어 시스템(부동 소수점 숫자의 영어 표현에서 소수점 자리에 쉼표가 사용됨)으로 숫자를 프린트하려면 다음을 사용합니다.
System.out.format(Locale.FRANCE,
"The value of the float " + "variable is %f, while the " +
"value of the integer variable " + "is %d, and the string is %s%n",
floatVar, intVar, stringVar);
An Example
다음 테이블에는 테이블 뒤에 나오는 샘플 프로그램 TestFormat.java에 사용되는 일부 컨버터 및 플래그가 나열되어 있습니다.
Converters and Flags Used in TestFormat.java
Converter | Flag | Explanation |
d | A decimal integer. | |
f | A float. | |
n | A new line character appropriate to the platform running the application. You should always use %n, rather than \n. | |
tB | A date & time conversion—locale-specific full name of month. | |
td, te | A date & time conversion—2-digit day of month. td has leading zeroes as needed, te does not. | |
ty, tY | A date & time conversion—ty = 2-digit year, tY = 4-digit year. | |
tl | A date & time conversion—hour in 12-hour clock. | |
tM | A date & time conversion—minutes in 2 digits, with leading zeroes as necessary. | |
tp | A date & time conversion—locale-specific am/pm (lower case). | |
tm | A date & time conversion—months in 2 digits, with leading zeroes as necessary. | |
tD | A date & time conversion—date as %tm%td%ty | |
08 | Eight characters in width, with leading zeroes as necessary. | |
+ | Includes sign, whether positive or negative. | |
, | Includes locale-specific grouping characters. | |
- | Left-justified.. | |
.3 | Three places after decimal point. | |
10.3 | Ten characters in width, right justified, with three places after decimal point. |
다음 프로그램은 format을 사용하여 수행할 수 있는 포맷화 중 일부를 보여줍니다. 출력은 포함된 주석에서 큰따옴표 안에 표시됩니다.
import java.util.Calendar;
import java.util.Locale;
public class TestFormat {
public static void main(String[] args) {
long n = 461012;
System.out.format("%d%n", n); // --> "461012"
System.out.format("%08d%n", n); // --> "00461012"
System.out.format("%+8d%n", n); // --> " +461012"
System.out.format("%,8d%n", n); // --> " 461,012"
System.out.format("%+,8d%n%n", n); // --> "+461,012"
double pi = Math.PI;
System.out.format("%f%n", pi); // --> "3.141593"
System.out.format("%.3f%n", pi); // --> "3.142"
System.out.format("%10.3f%n", pi); // --> " 3.142"
System.out.format("%-10.3f%n", pi); // --> "3.142"
System.out.format(Locale.FRANCE,
"%-10.4f%n%n", pi); // --> "3,1416"
Calendar c = Calendar.getInstance();
System.out.format("%tB %te, %tY%n", c, c, c); // --> "May 29, 2006"
System.out.format("%tl:%tM %tp%n", c, c, c); // --> "2:34 am"
System.out.format("%tD%n", c); // --> "05/29/06"
}
}
참고: 이 섹션의 논의에서는 형식 및 printf 메소드의 기본 사항만 다룹니다. 자세한 내용은 Essential Trail의 기본 I/O 섹션에 있는 "Formatting" 페이지에서 확인할 수 있습니다.
String.format을 사용하여 문자열을 만드는 방법은 Strings에서 다룹니다.
The DecimalFormat Class
생략
Beyond Basic Arithmetic
생략
Random Numbers
random() 메서드는 0.0과 1.0 사이에서 의사 무작위로 선택된 숫자를 반환합니다. 범위에는 0.0이 포함되지만 1.0은 포함되지 않습니다. 즉, 0.0 <= Math.random() < 1.0입니다. 다른 범위의 숫자를 얻으려면 무작위 방법으로 반환된 값에 대해 산술 연산을 수행할 수 있습니다. 예를 들어, 0에서 9 사이의 정수를 생성하려면 다음과 같이 작성합니다.
int number = (int)(Math.random() * 10);
해당 값에 10을 곱하면 가능한 값의 범위는 0.0 <= 숫자 < 10.0이 됩니다.
Math.random을 사용하면 단일 난수를 생성해야 할 때 효과적입니다. 일련의 난수를 생성해야 하는 경우 java.util.Random 인스턴스를 생성하고 해당 객체에 대한 메서드를 호출하여 숫자를 생성해야 합니다.
'High Level Programming Language > Learning the Java Language' 카테고리의 다른 글
Lesson: Numbers and Strings [Autoboxing and Unboxing] (0) | 2024.06.05 |
---|---|
Lesson: Numbers and Strings [Strings] (0) | 2024.06.05 |
Lesson: Interfaces and Inheritance[Polymorphism] (0) | 2024.06.04 |
Lesson: Interfaces and Inheritance[Inheritance] (0) | 2024.06.03 |
Lesson: Interfaces and Inheritance[Interfaces] (0) | 2024.06.03 |